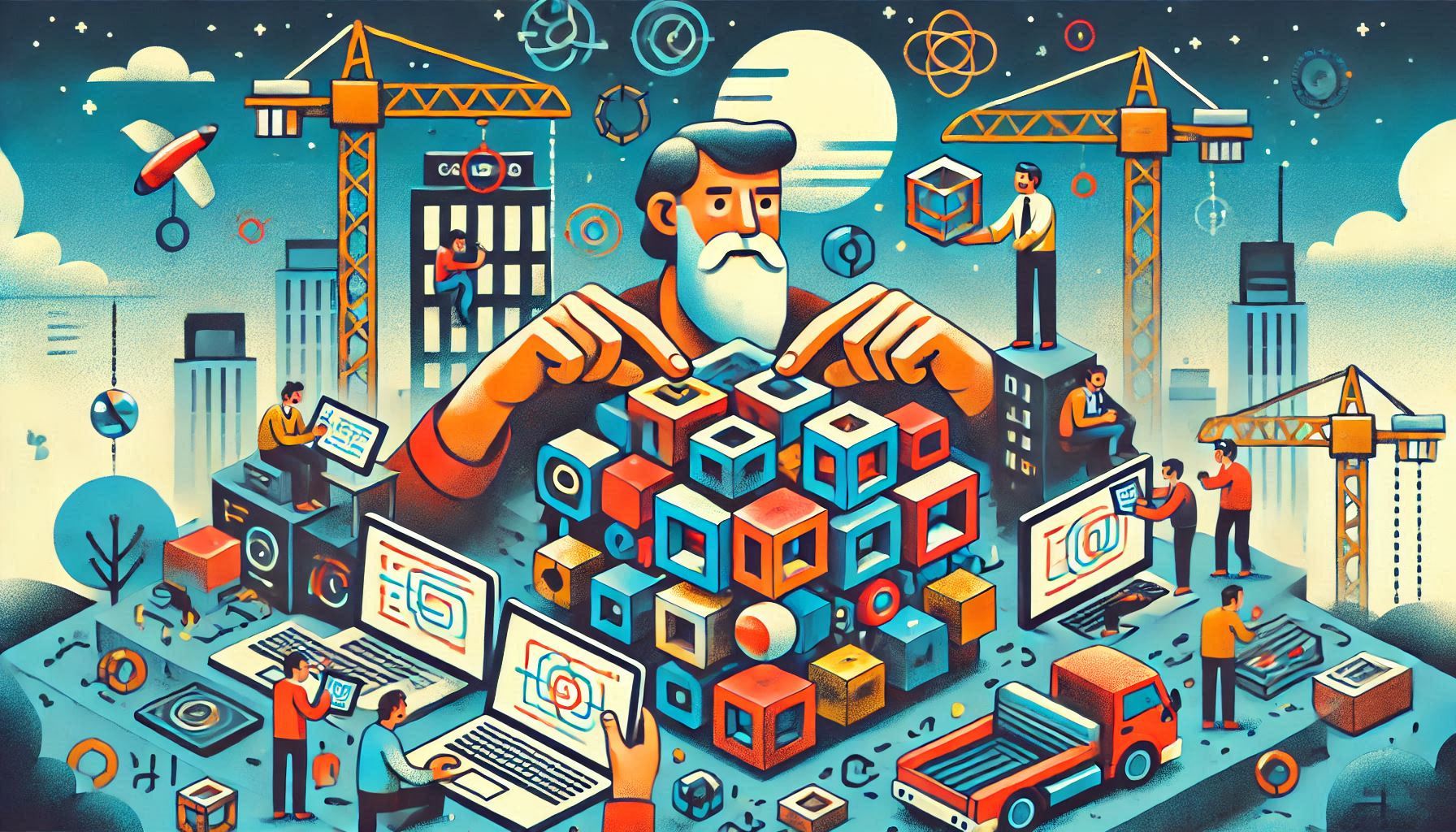
Understanding SOLID Principles for Maintainable and Flexible Object-Oriented Software Design
The SOLID principles are a set of five design principles that were introduced by Robert C. Martin to help software developers create more maintainable, flexible, and understandable software. These principles are guidelines that can be applied to object-oriented programming (OOP) to improve the overall quality of the code and make it easier to manage and extend. Here's a brief overview of each of the SOLID principles:
Single Responsibility Principle (SRP):
A class should have only one reason to change.
It means that a class should have only one responsibility or job.
When a class has multiple responsibilities, changes to one of those responsibilities can inadvertently affect the other responsibilities, making the code more fragile and harder to maintain.
Open/Closed Principle (OCP):
Software entities (classes, modules, functions, etc.) should be open for extension but closed for modification.
This principle encourages you to design your software in a way that allows you to add new features or functionality without changing the existing code.
You achieve this through techniques like inheritance, interfaces, and polymorphism.
Liskov Substitution Principle (LSP):
Subtypes (derived classes or derived types) must be substitutable for their base types without altering the correctness of the program.
In other words, objects of a derived class should be able to replace objects of the base class without affecting the program's behavior.
Violating this principle can lead to unexpected errors and bugs.
Interface Segregation Principle (ISP):
Clients should not be forced to depend on interfaces they do not use.
This principle suggests that you should have small, specific interfaces rather than large, monolithic ones.
It helps prevent clients from being burdened with unnecessary methods and dependencies.
Dependency Inversion Principle (DIP):
High-level modules should not depend on low-level modules. Both should depend on abstractions.
Abstractions should not depend on details. Details should depend on abstractions.
This principle promotes decoupling between high-level and low-level modules by introducing abstractions (interfaces or abstract classes) that both can depend on.
It allows for flexibility and easier substitution of components.
By adhering to the SOLID principles, developers can create software that is more maintainable, easier to extend, and less prone to bugs and unexpected behavior. These principles are fundamental to object-oriented design and are widely used in software development practices.
Single Responsibility Principle (SRP) UML example
The Single Responsibility Principle (SRP) is one of the SOLID principles in object-oriented programming. It states that a class should have only one reason to change, meaning that a class should have only one responsibility or job. To illustrate the SRP with a UML (Unified Modeling Language) example, let's consider a simple scenario involving a class responsible for logging messages.
Here's a UML class diagram that demonstrates the Single Responsibility Principle:
In this example:
Logger is a class responsible for logging messages.
It has the following attributes:
logFile: A reference to a log file where messages will be logged.
logToConsole: A boolean flag indicating whether logging to the console is enabled.
It has the following methods:
log(message: string): void: Logs the given message to both the log file and the console if enabled.
setLogFile(file: File): void: Sets the log file to a new file.
enableConsoleLogging(): void: Enables logging to the console.
disableConsoleLogging(): void: Disables logging to the console.
In this design, the Logger class has a single responsibility, which is to handle logging. It manages the log file and provides methods to log messages. It doesn't have unrelated responsibilities, such as formatting messages, parsing configuration files, or handling user authentication. By adhering to the SRP, this class is more maintainable and easier to understand, and changes to one aspect of logging (e.g., the log file or console logging) don't affect the other aspects of the class.
Separating concerns and ensuring that each class has a single responsibility is a fundamental principle in object-oriented design and helps improve code quality and maintainability.
Open/Closed Principle (OCP) UML example
The Open/Closed Principle (OCP) is one of the SOLID principles in object-oriented programming. It states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. In other words, you should be able to add new functionality to a module without changing its existing source code. To illustrate the OCP with a UML (Unified Modeling Language) example, let's consider a scenario involving shapes and area calculation.
Here's a UML class diagram that demonstrates the Open/Closed Principle:
In this example:
Shape is an abstract class that defines a method calculateArea() for calculating the area of a shape. This abstract class is open for extension, meaning you can create new shapes by inheriting from it.
Circle and Square are concrete classes that inherit from the Shape class. They provide their own implementations of the calculateArea() method specific to their shapes.
The OCP is demonstrated because you can easily extend this system to include new shapes (e.g., triangles, rectangles, or any other geometric shape) without modifying the existing code. You can simply create a new class that inherits from Shape and implements its own calculateArea() method for the new shape.
For example, adding a Triangle class:
You can add the Triangle class without changing the Shape, Circle, or Square classes, adhering to the Open/Closed Principle. This makes your code more maintainable and less prone to introducing bugs when you extend it with new functionality.
Liskov Substitution Principle (LSP) UML example
The Liskov Substitution Principle (LSP) is one of the SOLID principles in object-oriented programming. It states that objects of a derived class must be substitutable for objects of the base class without affecting the correctness of the program. In other words, if a class is a subtype of another class, it should be able to be used interchangeably with its base class without causing problems. To illustrate the LSP with a UML (Unified Modeling Language) example, let's consider a scenario involving shapes and area calculation.
Here's a UML class diagram that demonstrates the Liskov Substitution Principle:
In this example:
Shape is an abstract class that defines a method calculateArea() for calculating the area of a shape.
Circle and Square are concrete classes that inherit from the Shape class. They provide their own implementations of the calculateArea() method specific to their shapes.
The Liskov Substitution Principle is demonstrated because you can use objects of the derived classes (Circle and Square) interchangeably with objects of the base class (Shape) without affecting the correctness of the program. For example, you can write code like this:
Shape circle = new Circle(5.0);
Shape square = new Square(4.0);
double area1 = circle.calculateArea();
double area2 = square.calculateArea();
In this code, even though circle and square are declared as Shape, they are actually instances of Circle and Square, respectively. The LSP ensures that you can call calculateArea() on both circle and square, and each will correctly calculate its area based on its specific implementation.
By adhering to the Liskov Substitution Principle, your code remains robust and predictable when working with polymorphism and inheritance, as derived classes can seamlessly replace their base classes in any context where the base class is expected.
Interface Segregation Principle (ISP) UML example
The Interface Segregation Principle (ISP) is one of the SOLID principles in object-oriented programming. It states that clients should not be forced to depend on interfaces they do not use. In other words, an interface should have only the methods that are relevant to the clients that use it. To illustrate the ISP with a UML (Unified Modeling Language) example, let's consider a scenario involving a multifunctional printer.
Here's a UML class diagram that demonstrates the Interface Segregation Principle:
In this example:
Printer, Scanner, and FaxMachine are interfaces that define specific functionality: printing, scanning, and faxing, respectively.
MultiFunctionPrinter is a class that implements all three interfaces (Printer, Scanner, and FaxMachine) to create a multifunctional printer. This class provides implementations for all three methods: print(), scan(), and fax().
The ISP is demonstrated because clients that need only one specific functionality, such as printing or scanning, can depend on the respective interface without being forced to implement methods they do not use. For example, a client that only needs to print can depend on the Printer interface:
class Client {
private Printer printer;
public Client(Printer printer) {
this.printer = printer;
}
public void doPrint() {
printer.print();
}
}
In this code, the Client class depends on the Printer interface, allowing it to print without being concerned about scanning or faxing.
By adhering to the Interface Segregation Principle, you create more granular and focused interfaces, making it easier for clients to depend on only the functionality they require, thus reducing unnecessary dependencies and ensuring that clients are not forced to implement or rely on methods they don't need.
Dependency Inversion Principle (DIP) UML example
The Dependency Inversion Principle (DIP) is one of the SOLID principles in object-oriented programming. It states that high-level modules should not depend on low-level modules, but both should depend on abstractions. Abstractions should not depend on details; details should depend on abstractions. To illustrate this principle with a UML (Unified Modeling Language) example, let's consider a simple scenario involving a light switch.
Here's a UML class diagram that demonstrates the Dependency Inversion Principle:
In this example:
Switchable is an abstraction (interface or abstract class) that defines the contract for anything that can be turned on and off.
Light is a concrete implementation of the Switchable interface, representing a specific device that can be controlled.
LightSwitch is a high-level module that interacts with Switchable (abstraction) rather than directly with Light (low-level module).
The LightSwitch class depends on the Switchable abstraction, which follows the Dependency Inversion Principle. This allows you to easily switch to other implementations of Switchable without modifying the LightSwitch class. For example, if you wanted to control a different type of device, like a fan or a heater, you could create new classes that implement the Switchable interface and use them with the same LightSwitch class without changing its code.
By adhering to the DIP, you achieve a more flexible and maintainable design, as high-level modules depend on abstractions, making it easier to extend and replace components as needed without causing ripple effects throughout the codebase.