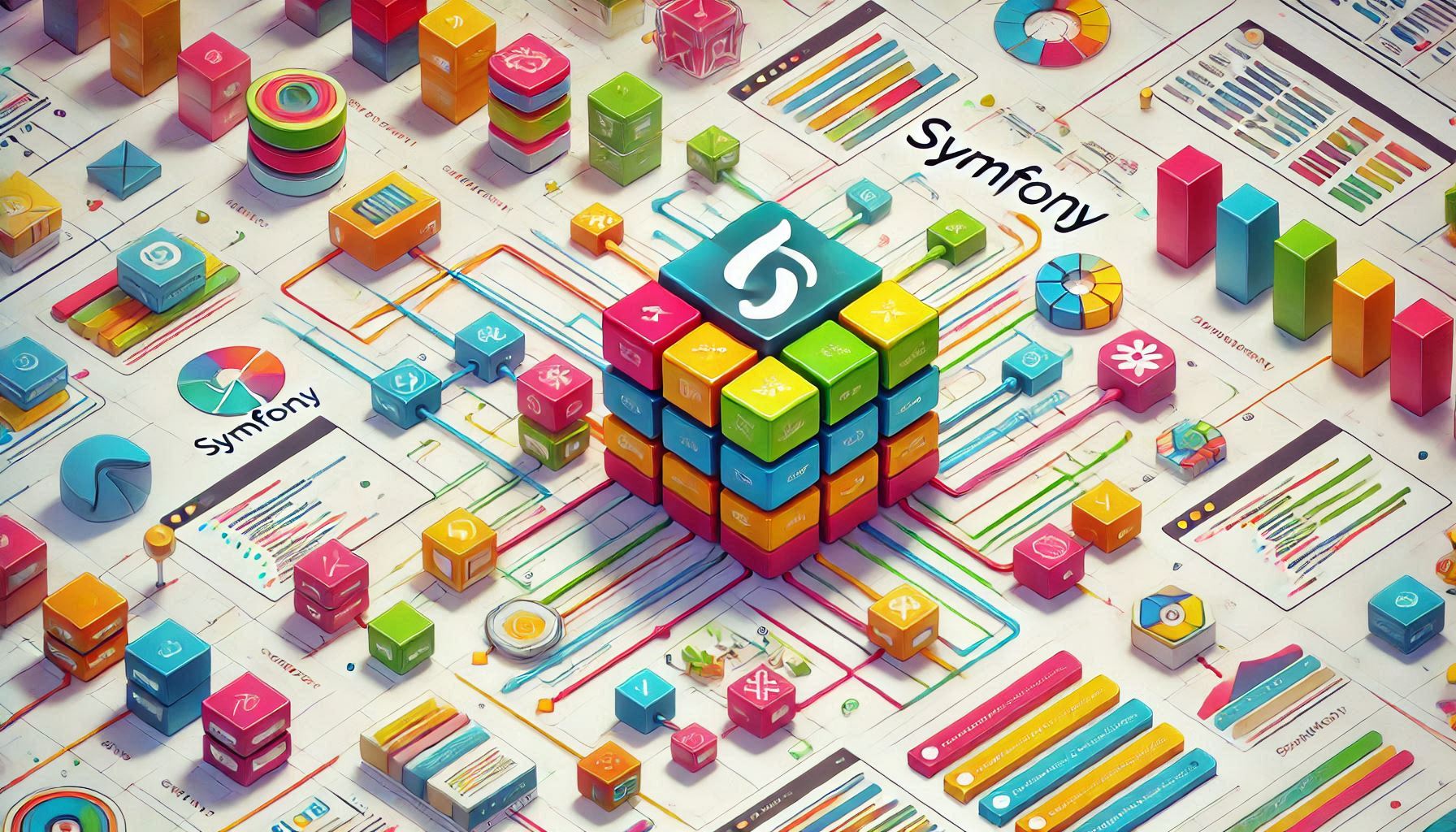
Architecting Symfony: Building Scalable Web Apps with Microservices, Events, Layered or Service Oriented Architectures
Web application architectures can be categorized into three fundamental architectural styles:
Pipe-and-Filter Architecture:
This architectural approach involves passing data through a sequence of processing components, known as filters, where each component incrementally transforms the data. Although strictly defined Pipe-and-Filter systems may be less common in modern web applications, the concept finds relevance in certain architectures:
Microservices Architecture: While not a pure implementation of Pipe-and-Filter, microservices often apply this pattern within individual services or as part of broader data processing pipelines.
Event-Based Architecture:
Event-based architecture relies heavily on events to coordinate communication and trigger actions between decoupled components or services, promoting a highly flexible and responsive system design. Notable examples include:
Microservices Architecture: Typically favors event-driven communication, especially for interactions across different services.
Serverless Architecture: Frequently event-driven, with functions activated by diverse events such as HTTP requests, database changes, or message queues.
Event-Driven Architecture: Naturally aligns with this category, where events serve as the primary communication mechanism.
Layered Architecture:
This style organizes a system into distinct layers, each with specific roles and responsibilities, often arranged in a hierarchical manner. Examples include:
Layered (N-Tier) Architecture: Directly associated with this category, commonly featuring layers such as presentation, business logic, and data.
Service-Oriented Architecture (SOA): Though SOA can incorporate event-driven components, it often employs a layered arrangement to structure services based on functionality, achieving a clear separation of concerns across different tiers.
Each of these architectural styles has its unique characteristics and is selected based on the specific needs and goals of a web application, influencing factors such as scalability, maintainability, and flexibility.
Microservices Architecture
Here's an outline of folders and files for a web application implementing a Microservices Architecture using Symfony. Each microservice will have its own directory structure and will be treated as an individual Symfony project.
web-app/
│
├── auth-service/
│ ├── config/
│ ├── src/
│ │ ├── Controller/
│ │ ├── Entity/
│ │ ├── Repository/
│ │ ├── Service/
│ │ └── Kernel.php
│ ├── templates/
│ ├── tests/
│ ├── var/
│ ├── public/
│ ├── bin/
│ ├── docker/
│ │ ├── Dockerfile
│ ├── docker-compose.yml
│ ├── composer.json
│ └── README.md
│
├── user-service/
│ ├── config/
│ ├── src/
│ │ ├── Controller/
│ │ ├── Entity/
│ │ ├── Repository/
│ │ ├── Service/
│ │ └── Kernel.php
│ ├── templates/
│ ├── tests/
│ ├── var/
│ ├── public/
│ ├── bin/
│ ├── docker/
│ │ ├── Dockerfile
│ ├── docker-compose.yml
│ ├── composer.json
│ └── README.md
│
├── common/
│ ├── config/
│ ├── src/
│ │ ├── Util/
│ │ ├── Event/
│ │ └── Exception/
│ ├── tests/
│ └── README.md
│
├── README.md
└── .gitignore
user-service/config/
Configuration files such as services, routes, and packages.
user-service/
└── config/
├── packages/
│ ├── all.yaml
│ ├── doctrine.yaml
│ └── security.yaml
├── routes/
│ ├── annotations.yaml
│ └── routes.yaml
├── services.yaml
└── bundles.php
ser-service/src/Controller/
Handles HTTP requests and returns responses.
user-service/
└── src/
└── Controller/
├── UserController.php
user-service/src/Entity/
Defines data models and ORM entities.
user-service/
└── src/
└── Entity/
├── User.php
user-service/src/Repository/
Handles database interactions.
user-service/
└── src/
└── Repository/
├── UserRepository.php
user-service/src/Service/
Contains the business logic of the application.
user-service/
└── src/
└── Service/
├── UserService.php
user-service/src/
Entry point of the service.
user-service/
└── src/
└── Kernel.php
user-service/templates/
Contains Twig templates for rendering views.
user-service/
└── templates/
└── user/
├── profile.html.twig
user-service/tests/
Contains test cases for the service.
user-service/
└── tests/
├── Controller/
│ ├── UserControllerTest.php
├── Service/
│ ├── UserServiceTest.php
Root-Level Files for user-service
Dockerfile: Defines how to containerize the microservice.
composer.json: Contains metadata and dependencies for the service.
README.md: Documentation for the service.
user-service/
├── docker/
│ ├── Dockerfile
├── docker-compose.yml
├── composer.json
└── README.md
common/ Directory
Contains shared utilities, configuration files, events, and exceptions used across multiple services.
common/
├── config/
│ ├── common.yaml
├── src/
│ ├── Util/
│ │ ├── Logger.php
│ │ └── Validator.php
│ ├── Event/
│ │ ├── UserRegisteredEvent.php
│ ├── Exception/
│ │ ├── CustomException.php
├── tests/
│ ├── Util/
│ │ ├── LoggerTest.php
│ │ └── ValidatorTest.php
└── README.md
Event-Driven Architecture
Implementing an Event-Driven Architecture in a Symfony web application involves structuring the application so that components communicate primarily through events. This structure typically includes event dispatchers, listeners, and handlers. Here's an example of a folder and file structure for a Symfony web application designed around an Event-Driven Architecture.
web-app/
│
├── config/
│ ├── packages/
│ ├── routes/
│ ├── services.yaml
│ ├── bundles.php
│ └── messenger.yaml
│
├── src/
│ ├── Controller/
│ ├── Entity/
│ ├── Event/
│ ├── EventListener/
│ ├── EventSubscriber/
│ ├── Repository/
│ ├── Service/
│ ├── Message/
│ ├── MessageHandler/
│ └── Kernel.php
│
├── templates/
│
├── tests/
│ ├── Controller/
│ ├── Service/
│ ├── Event/
│ ├── EventListener/
│ ├── EventSubscriber/
│ └── MessageHandler/
│
├── var/
│
├── public/
│
├── bin/
│
├── vendor/
│
├── .env
├── composer.json
├── symfony.lock
├── README.md
└── .gitignore
config/
Configuration files for Symfony framework, including service definitions and routing.
config/
├── packages/
│ ├── all.yaml
│ ├── doctrine.yaml
│ ├── framework.yaml
│ ├── messenger.yaml # Configuration for Symfony Messenger component
│ ├── security.yaml
│ ├── services.yaml
│ └── bundles.php
├── routes/
│ ├── annotations.yaml
│ └── routes.yaml
├── services.yaml
└── bundles.php
src/Controller/
Handles incoming HTTP requests and returns responses.
src/
└── Controller/
├── UserController.php
├── OrderController.php
src/Entity/
Defines data models and ORM entities.
src/
└── Entity/
├── User.php
├── Order.php
src/Event/
Defines custom events for the application.
src/
└── Event/
├── UserRegisteredEvent.php
├── OrderPlacedEvent.php
src/EventListener/
Contains event listeners that handle events.
src/
└── EventListener/
├── UserRegisteredListener.php
├── OrderPlacedListener.php
src/EventSubscriber/
Contains event subscribers that listen to multiple events.
src/
└── EventSubscriber/
├── UserEventSubscriber.php
├── OrderEventSubscriber.php
src/Repository/
Handles database interactions.
src/
└── Repository/
├── UserRepository.php
├── OrderRepository.php
src/Service/
Contains business logic and service classes.
src/
└── Service/
├── UserService.php
├── OrderService.php
src/Message/
Defines messages for asynchronous processing.
src/
└── Message/
├── UserRegisteredMessage.php
├── OrderPlacedMessage.php
src/MessageHandler/
Handles processing of messages.
src/
└── MessageHandler/
├── UserRegisteredMessageHandler.php
├── OrderPlacedMessageHandler.php
src/
Entry point of the service.
src/
└── Kernel.php
templates/
Contains Twig templates for rendering views.
templates/
└── user/
├── profile.html.twig
└── order/
├── confirmation.html.twig
tests/
Contains test cases for the application.
tests/
└── Controller/
├── UserControllerTest.php
├── OrderControllerTest.php
└── Service/
├── UserServiceTest.php
├── OrderServiceTest.php
└── Event/
├── UserRegisteredEventTest.php
├── OrderPlacedEventTest.php
└── EventListener/
├── UserRegisteredListenerTest.php
├── OrderPlacedListenerTest.php
└── EventSubscriber/
├── UserEventSubscriberTest.php
├── OrderEventSubscriberTest.php
└── MessageHandler/
├── UserRegisteredMessageHandlerTest.php
├── OrderPlacedMessageHandlerTest.php
Root-Level Files
.env: Environment configuration.
composer.json: Contains metadata and dependencies for the application.
README.md: Documentation for the project.
.gitignore: Specifies files and directories to be ignored by Git.
web-app/
├── .env
├── composer.json
├── symfony.lock
├── README.md
└── .gitignore
This structure ensures that the application is organized around events, with clear separation of concerns. Events can trigger various actions across different parts of the system, making the architecture scalable and maintainable.
Layered (N-Tier) Architecture
Implementing a Layered (N-Tier) Architecture in a Symfony web application involves organizing the application into distinct layers, each responsible for a specific part of the application's functionality. These layers typically include the presentation layer, the business logic layer, the data access layer, and the infrastructure layer. Here's an example of a folder and file structure for a Symfony web application designed around a Layered Architecture.
Root Directory Structure
web-app/
├── config/
│ ├── packages/
│ ├── routes/
│ ├── services.yaml
│ ├── bundles.php
│ └── routes.yaml
│
├── src/
│ ├── Controller/ # Presentation Layer
│ ├── Service/ # Business Logic Layer
│ ├── Repository/ # Data Access Layer
│ ├── Entity/ # Domain Layer
│ ├── Form/ # Presentation Layer (Forms)
│ ├── Security/ # Infrastructure Layer (Security)
│ ├── EventListener/ # Infrastructure Layer (Event Listeners)
│ ├── Migrations/ # Infrastructure Layer (Database Migrations)
│ ├── Kernel.php # Entry point
│
├── templates/ # Presentation Layer (Views)
│
├── tests/
│ ├── Controller/
│ ├── Service/
│ ├── Repository/
│ └── Entity/
│
├── var/
│
├── public/
│
├── bin/
│
├── vendor/
│
├── .env
├── composer.json
├── symfony.lock
├── README.md
└── .gitignore
config/
Configuration files for Symfony framework, including service definitions and routing.
config/
├── packages/
│ ├── all.yaml
│ ├── doctrine.yaml
│ ├── framework.yaml
│ ├── security.yaml
│ └── services.yaml
├── routes/
│ ├── annotations.yaml
│ ├── routes.yaml
├── bundles.php
└── routes.yaml
src/Controller/ (Presentation Layer)
Handles incoming HTTP requests and returns responses.
src/
└── Controller/
├── UserController.php
├── OrderController.php
src/Service/ (Business Logic Layer)
Contains the business logic and service classes.
src/
└── Service/
├── UserService.php
├── OrderService.php
src/Repository/ (Data Access Layer)
Handles database interactions.
src/
└── Repository/
├── UserRepository.php
├── OrderRepository.php
src/Entity/ (Domain Layer)
Defines data models and ORM entities.
src/
└── Entity/
├── User.php
├── Order.php
src/Form/ (Presentation Layer - Forms)
Handles form creation and validation.
src/
└── Form/
├── UserType.php
├── OrderType.php
src/Security/ (Infrastructure Layer)
Contains security-related classes, like user providers and Voters.
src/
└── Security/
├── UserProvider.php
├── Voter/
│ ├── UserVoter.php
src/EventListener/ (Infrastructure Layer)
Contains event listeners that handle system events.
src/
└── EventListener/
├── UserRegisteredListener.php
├── OrderPlacedListener.php
src/Migrations/ (Infrastructure Layer)
Contains database migration files.
src/
└── Migrations/
├── Version20210601000000.php
├── Version20210701000000.php
src/ (Entry Point)
Entry point of the service
src/
└── Kernel.php
templates/ (Presentation Layer)
Contains Twig templates for rendering views.
templates/
└── user/
├── profile.html.twig
└── order/
├── confirmation.html.twig
tests/
Contains test cases for the application.
tests/
└── Controller/
├── UserControllerTest.php
├── OrderControllerTest.php
└── Service/
├── UserServiceTest.php
├── OrderServiceTest.php
└── Repository/
├── UserRepositoryTest.php
├── OrderRepositoryTest.php
└── Entity/
├── UserTest.php
├── OrderTest.php
This structure ensures clear separation of concerns, making the application easier to develop, test, and maintain. Each layer has its own responsibility, and changes in one layer have minimal impact on others.
Service-Oriented Architecture (SOA)
Implementing a Service-Oriented Architecture (SOA) in a Symfony web application involves structuring the application around distinct services that communicate through well-defined interfaces. Each service is self-contained and responsible for a specific part of the application's functionality. Here's an example of a folder and file structure for a Symfony web application designed around a Service-Oriented Architecture.
Root Directory Structure
web-app/
│
├── services/
│ ├── user-service/
│ ├── order-service/
│ ├── payment-service/
│ └── common/
│
├── config/
│ ├── packages/
│ ├── routes/
│ ├── services.yaml
│ ├── bundles.php
│ └── routes.yaml
│
├── src/
│ ├── Controller/
│ ├── EventListener/
│ ├── Migrations/
│ ├── Security/
│ ├── Kernel.php
│
├── templates/
│
├── tests/
│
├── var/
│
├── public/
│
├── bin/
│
├── vendor/
│
├── .env
├── composer.json
├── symfony.lock
├── README.md
└── .gitignore
Service Directory Structure (Example: `user-service`)
Each service has its own directory, containing its own configuration, controllers, entities, repositories, services, and templates.
`services/user-service/`
user-service/
├── config/
│ ├── packages/
│ ├── routes/
│ ├── services.yaml
│ ├── bundles.php
│ └── routes.yaml
│
├── src/
│ ├── Controller/
│ ├── Entity/
│ ├── Repository/
│ ├── Service/
│ ├── Form/
│ ├── Event/
│ ├── EventListener/
│ └── Kernel.php
│
├── templates/
│ ├── user/
│ ├── profile.html.twig
│ ├── new.html.twig
│
├── tests/
│ ├── Controller/
│ ├── Service/
│ ├── Repository/
│ └── Entity/
│
├── .env
├── composer.json
├── symfony.lock
├── README.md
└── .gitignore
Detailed Directory Structure for `user-service`
`config/`
- Configuration files specific to the `user-service`.
config/
├── packages/
│ ├── all.yaml
│ ├── doctrine.yaml
│ ├── framework.yaml
│ ├── security.yaml
│ └── services.yaml
├── routes/
│ ├── annotations.yaml
│ ├── routes.yaml
├── services.yaml
└── bundles.php
`src/Controller/`
- Handles incoming HTTP requests and returns responses.
src/
└── Controller/
├── UserController.php
`src/Entity/`
- Defines data models and ORM entities.
src/
└── Entity/
├── User.php
`src/Repository/`
- Handles database interactions.
src/
└── Repository/
├── UserRepository.php
`src/Service/`
- Contains the business logic and service classes.
src/
└── Service/
├── UserService.php
`src/Form/`
- Handles form creation and validation.
src/
└── Form/
├── UserType.php
```
`src/Event/`
- Defines custom events.
src/
└── Event/
├── UserRegisteredEvent.php
`src/EventListener/`
- Contains event listeners that handle events.
src/
└── EventListener/
├── UserRegisteredListener.php
`src/`
- Entry point of the service.
src/
└── Kernel.php
`templates/`
- Contains Twig templates for rendering views.
templates/
└── user/
├── profile.html.twig
├── new.html.twig
`tests/`
- Contains test cases for the service.
tests/
└── Controller/
├── UserControllerTest.php
└── Service/
├── UserServiceTest.php
└── Repository/
├── UserRepositoryTest.php
└── Entity/
├── UserTest.php
Root-Level Files for `user-service`
- `.env`: Environment configuration.
- `composer.json`: Contains metadata and dependencies for the service.
- `README.md`: Documentation for the service.
- `.gitignore`: Specifies files and directories to be ignored by Git.
user-service/
├── .env
├── composer.json
├── symfony.lock
├── README.md
└── .gitignore
Common Directory for Shared Components (`services/common/`)
- Contains shared utilities, configuration files, events, and exceptions used across multiple services.
common/
├── config/
│ ├── common.yaml
├── src/
│ ├── Util/
│ │ ├── Logger.php
│ │ └── Validator.php
│ ├── Event/
│ │ ├── UserRegisteredEvent.php
│ ├── Exception/
│ │ ├── CustomException.php
├── tests/
│ ├── Util/
│ │ ├── LoggerTest.php
│ │ └── ValidatorTest.php
└── README.md
Root-Level Files for the Entire Project
- `.env`: Environment configuration.
- `composer.json`: Contains metadata and dependencies for the application.
- `README.md`: Documentation for the project.
- `.gitignore`: Specifies files and directories to be ignored by Git.
web-app/
├── .env
├── composer.json
├── symfony.lock
├── README.md
└── .gitignore
This structure ensures clear separation of concerns and modularity, making the application easier to develop, test, and maintain. Each service is self-contained and can be developed, deployed, and scaled independently.